17. Functions
Functions
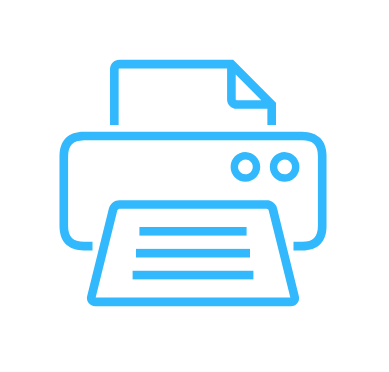
The last thing you will need to learn in order to complete the next exercise is how to write a function. Fortunately, you have seen a function before when you wrote
main()
!
When a function is declared and defined in a single C++ file, the basic syntax is as follows:
return_type FunctionName(parameter_list) {
// Body of function here.
}
See the following notebook for examples:
Workspace
This section contains either a workspace (it can be a Jupyter Notebook workspace or an online code editor work space, etc.) and it cannot be automatically downloaded to be generated here. Please access the classroom with your account and manually download the workspace to your local machine. Note that for some courses, Udacity upload the workspace files onto https://github.com/udacity , so you may be able to download them there.
Workspace Information:
- Default file path:
- Workspace type: jupyter
- Opened files (when workspace is loaded): n/a
On to the Exercise
Excellent work! You have learned quite a lot in the last few concepts, including:
- Accessing elements of a vector and getting the vector's size.
-
How
for
loops work in C++, using iterators and range-based loops. - Increment (and decrement) operators.
- How to write your own functions.
In the next exercise, you will write two
for
loops to print the contents of a 2D vector so you will be able to print the grid in your project!