29. Great Work!
Great Work!
C++ND C1 L02 Outro
Summary
Great work! You now have a C++ program which reads board data, stores the board in your program, and prints the board with nice formatting. You are ready to begin writing the A* search function.
Here is the outline summarizing what you have learned in this lesson:
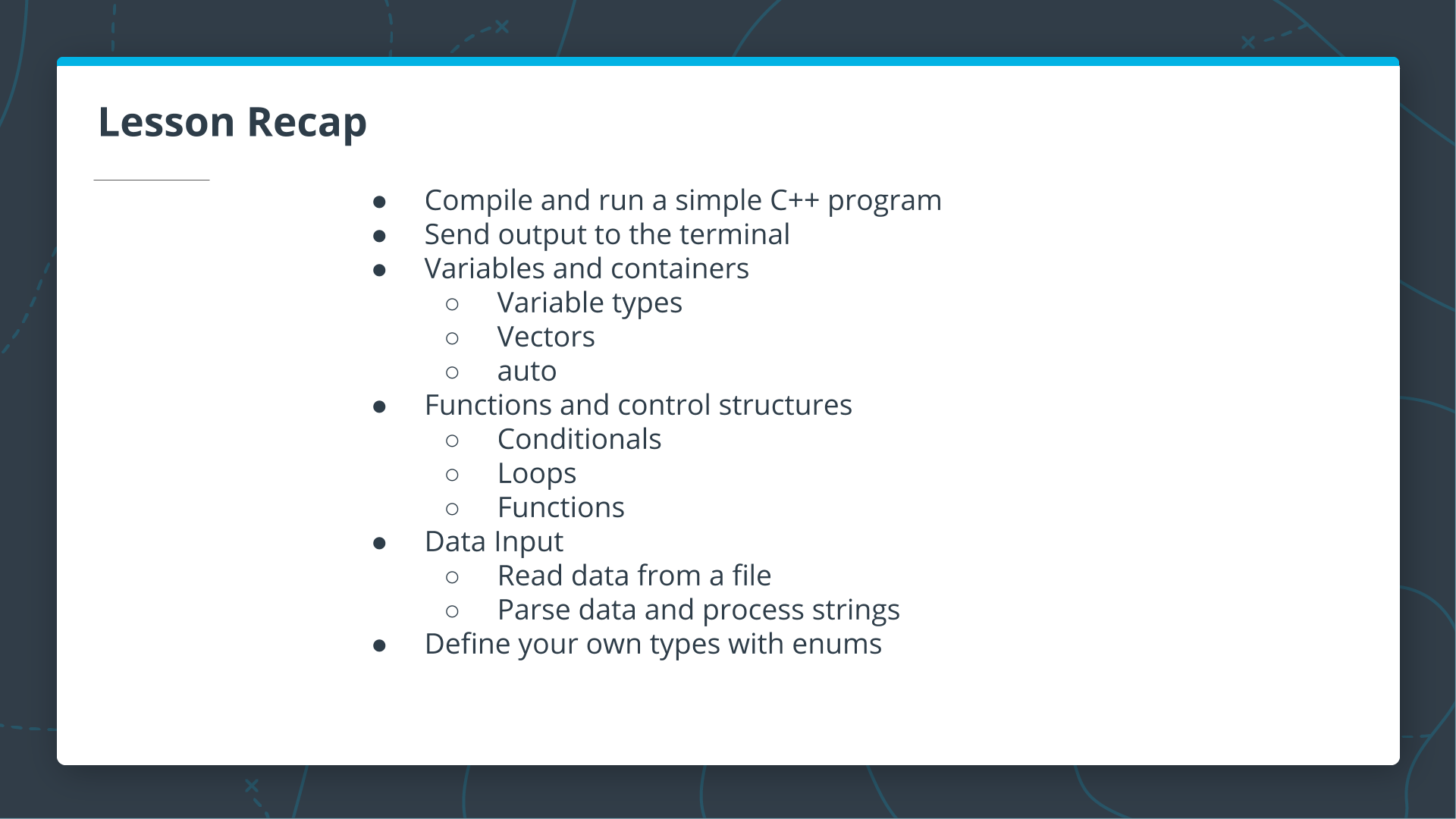
Review
At this point, your program should look like the program below. You have built this short program from scratch, so now is great time to review the code to ensure you recall how each part of it works.
#include <fstream>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cout;
using std::ifstream;
using std::istringstream;
using std::string;
using std::vector;
enum class State {kEmpty, kObstacle};
vector<State> ParseLine(string line) {
istringstream sline(line);
int n;
char c;
vector<State> row;
while (sline >> n >> c && c == ',') {
if (n == 0) {
row.push_back(State::kEmpty);
} else {
row.push_back(State::kObstacle);
}
}
return row;
}
vector<vector<State>> ReadBoardFile(string path) {
ifstream myfile (path);
vector<vector<State>> board{};
if (myfile) {
string line;
while (getline(myfile, line)) {
vector<State> row = ParseLine(line);
board.push_back(row);
}
}
return board;
}
string CellString(State cell) {
switch(cell) {
case State::kObstacle: return "⛰️ ";
default: return "0 ";
}
}
void PrintBoard(const vector<vector<State>> board) {
for (int i = 0; i < board.size(); i++) {
for (int j = 0; j < board[i].size(); j++) {
cout << CellString(board[i][j]);
}
cout << "\n";
}
}
int main() {
auto board = ReadBoardFile("1.board");
PrintBoard(board);
}